-->
Describes an object that controls a sequence of length N
of elements of type Ty
. The sequence is stored as an array of Ty
, contained in the array<Ty, N>
object.
除了begin和end之外,cbegin和cend不是std:: initializerlist的接口的一部分,我无法提供一个洞察,所以为什么最后两个成员函数应该在那里. The range-based for loop never uses cbegin or cend. (Therefore there is no way to force it.) There are surprisingly many rumors to the contrary; some believe that cbegin and cend are used, but never try whether the same code would compile without begin and end.A trivial example follows.
Syntax
Parameters
Parameter | Description |
---|---|
Ty | The type of an element. |
N | The number of elements. |
Members
Type Definition | Description |
---|---|
const_iterator | The type of a constant iterator for the controlled sequence. |
const_pointer | The type of a constant pointer to an element. |
const_reference | The type of a constant reference to an element. |
const_reverse_iterator | The type of a constant reverse iterator for the controlled sequence. |
difference_type | The type of a signed distance between two elements. |
iterator | The type of an iterator for the controlled sequence. |
pointer | The type of a pointer to an element. |
reference | The type of a reference to an element. |
reverse_iterator | The type of a reverse iterator for the controlled sequence. |
size_type | The type of an unsigned distance between two elements. |
value_type | The type of an element. |
Member Function | Description |
---|---|
array | Constructs an array object. |
assign | (Obsolete. Use fill .) Replaces all elements. |
at | Accesses an element at a specified position. |
back | Accesses the last element. |
begin | Designates the beginning of the controlled sequence. |
cbegin | Returns a random-access const iterator to the first element in the array. |
cend | Returns a random-access const iterator that points just beyond the end of the array. |
crbegin | Returns a const iterator to the first element in a reversed array. |
crend | Returns a const iterator to the end of a reversed array. |
data | Gets the address of the first element. |
empty | Tests whether elements are present. |
end | Designates the end of the controlled sequence. |
fill | Replaces all elements with a specified value. |
front | Accesses the first element. |
max_size | Counts the number of elements. |
rbegin | Designates the beginning of the reversed controlled sequence. |
rend | Designates the end of the reversed controlled sequence. |
size | Counts the number of elements. |
swap | Swaps the contents of two containers. |
Cbegin Cend
Operator | Description |
---|---|
array::operator= | Replaces the controlled sequence. |
array::operator[] | Accesses an element at a specified position. |
Remarks
The type has a default constructor array()
and a default assignment operator operator=
, and satisfies the requirements for an aggregate
. Therefore, objects of type array<Ty, N>
can be initialized by using an aggregate initializer. For example,
creates the object ai
that holds four integer values, initializes the first three elements to the values 1, 2, and 3, respectively, and initializes the fourth element to 0.
Requirements
Header: <array>
Namespace: std
array::array
Constructs an array object.
Parameters
right
Object or range to insert.
Remarks
The default constructor array()
leaves the controlled sequence uninitialized (or default initialized). You use it to specify an uninitialized controlled sequence.
The copy constructor array(const array& right)
initializes the controlled sequence with the sequence [right.begin()
, right.end()
). You use it to specify an initial controlled sequence that is a copy of the sequence controlled by the array object right.
Example
array::assign
Obsolete in C++11, replaced by fill. Replaces all elements.
array::at
Accesses an element at a specified position.
Parameters
off
Position of element to access.
Remarks
The member functions return a reference to the element of the controlled sequence at position off. If that position is invalid, the function throws an object of class out_of_range
.
Example
array::back
Accesses the last element.
Remarks
The member functions return a reference to the last element of the controlled sequence, which must be non-empty.
Example
array::begin
Designates the beginning of the controlled sequence.
Remarks
The member functions return a random-access iterator that points at the first element of the sequence (or just beyond the end of an empty sequence).
Home of the Auto-Tune plug-in, the music industry standard for pitch correction and vocal effects. Shop and learn about the best plug-ins for pitch correction, vocal effects, voice processing, and noise reduction. Auto-Tune Pro, Auto-Tune Artist, Auto-Tune EFX+, Auto-Tune. Antares auto tune vocal.
https://docuclever947.weebly.com/blog/spotify-premium-with-free-alexa. There’s also Premium for Family, which gives access to up to six people living at the same address. This is known as Spotify Premium.Premium is cheaper for students.
Example
array::cbegin
Returns a const iterator that addresses the first element in the range.
Return Value
A const random-access iterator that points at the first element of the range, or the location just beyond the end of an empty range (for an empty range, cbegin() cend()
).
Remarks
With the return value of cbegin
, the elements in the range cannot be modified.
You can use this member function in place of the begin()
member function to guarantee that the return value is const_iterator
. Typically, it's used in conjunction with the auto type deduction keyword, as shown in the following example. In the example, consider Container
to be a modifiable (non- const) container of any kind that supports begin()
and cbegin()
.
array::cend
Returns a const iterator that addresses the location just beyond the last element in a range.
Return Value
A random-access iterator that points just beyond the end of the range.
Remarks
cend
is used to test whether an iterator has passed the end of its range.
You can use this member function in place of the end()
member function to guarantee that the return value is const_iterator
. Typically, it's used in conjunction with the auto type deduction keyword, as shown in the following example. In the example, consider Container
to be a modifiable (non- const) container of any kind that supports end()
and cend()
.
The value returned by cend
should not be dereferenced.
array::const_iterator
The type of a constant iterator for the controlled sequence.
Remarks
The type describes an object that can serve as a constant random-access iterator for the controlled sequence.
Example
array::const_pointer
The type of a constant pointer to an element.
Remarks
The type describes an object that can serve as a constant pointer to elements of the sequence.
Example
array::const_reference
The type of a constant reference to an element.
Remarks
The type describes an object that can serve as a constant reference to an element of the controlled sequence.
Example
array::const_reverse_iterator
The type of a constant reverse iterator for the controlled sequence.
Remarks
The type describes an object that can serve as a constant reverse iterator for the controlled sequence.
Example
array::crbegin
Free audio effects plugins. Returns a const iterator to the first element in a reversed array.
Return Value
A const reverse random-access iterator addressing the first element in a reversed array or addressing what had been the last element in the unreversed array.
Remarks
With the return value of crbegin
, the array object cannot be modified.
Example
array::crend
Little snitch discount. Returns a const iterator that addresses the location succeeding the last element in a reversed array. Cannot download photos from iphone 7plus to macbook.
Return Value
A const reverse random-access iterator that addresses the location succeeding the last element in a reversed array (the location that had preceded the first element in the unreversed array).
Remarks
crend
is used with a reversed array just as array::cend is used with a array.
With the return value of crend
(suitably decremented), the array object cannot be modified.
crend
can be used to test to whether a reverse iterator has reached the end of its array.
The value returned by crend
should not be dereferenced.
Example
array::data
Gets the address of the first element.
Remarks
The member functions return the address of the first element in the controlled sequence.
Example
array::difference_type
The type of a signed distance between two elements.
Remarks
The signed integer type describes an object that can represent the difference between the addresses of any two elements in the controlled sequence. It is a synonym for the type std::ptrdiff_t
.
Example
array::empty
Spotify change windows app download. Tests whether no elements are present.
Remarks
The member function returns true only if N 0
.
Example
array::end
Designates the end of the controlled sequence.
Remarks
The member functions return a random-access iterator that points just beyond the end of the sequence.
Example
array::fill
Erases a array and copies the specified elements to the empty array.
Parameters
Parameter | Description |
---|---|
val | The value of the element being inserted into the array. |
Remarks
fill
replaces each element of the array with the specified value.
Example
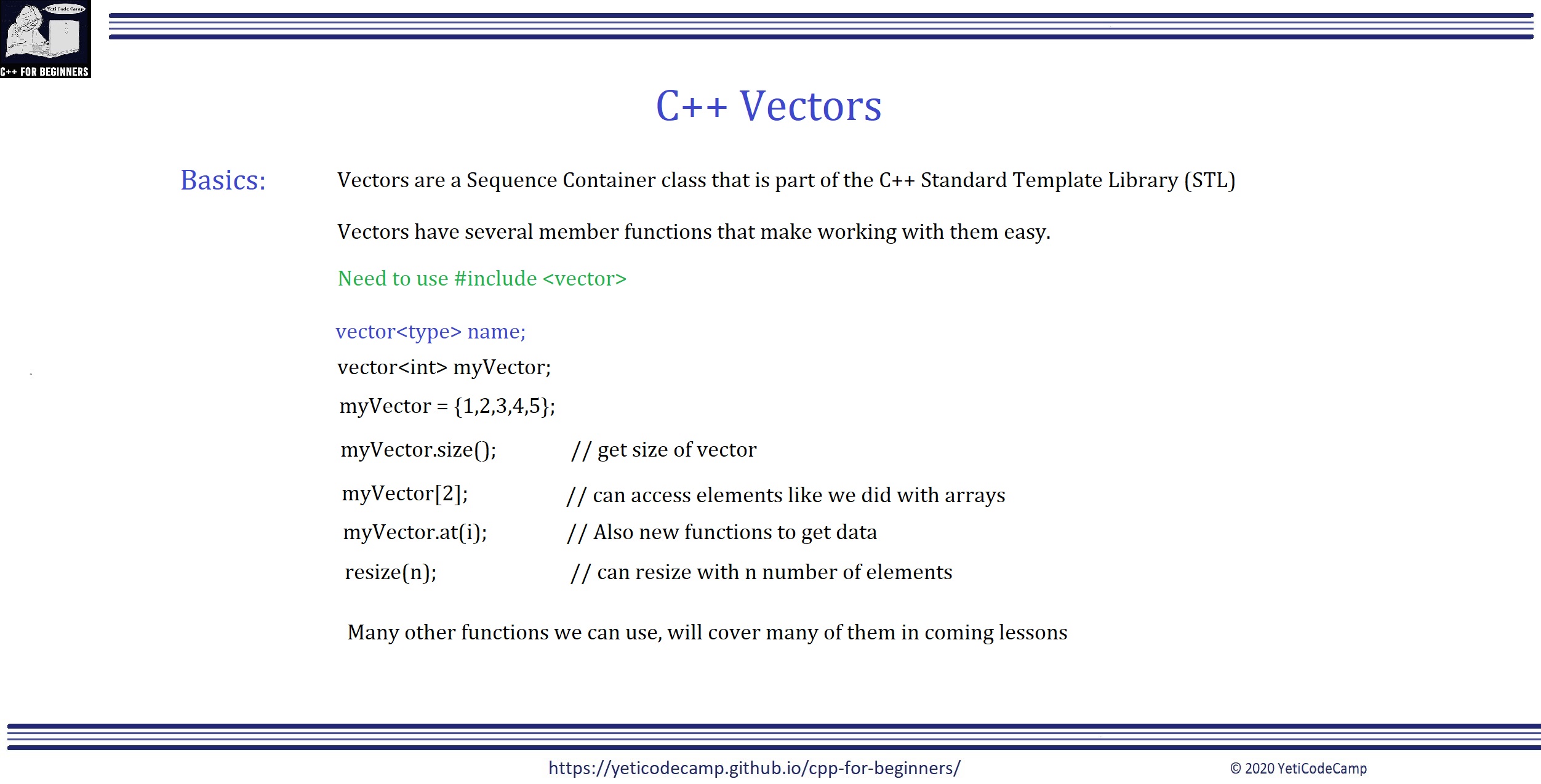
Cbegin Cend Dev C 5
array::front
Accesses the first element.
Remarks
The member functions return a reference to the first element of the controlled sequence, which must be non-empty.
Example
array::iterator
The type of an iterator for the controlled sequence. Sam cooke a change is gonna come cd download for windows 7.
Remarks
The type describes an object that can serve as a random-access iterator for the controlled sequence.
Example
array::max_size
Counts the number of elements.
Remarks
The member function returns N
.
Example
array::operator[]
Accesses an element at a specified position.
Parameters

off
Position of element to access.
Night light. https://localheavenly.weebly.com/blog/homedics-cool-mist-humidifier-user-manual. How is this possible? Warm or cool mists. Anti-bacteria tank. 2 demineralized cartridgeThis in house humidity controlling appliance like said earlier, it can be used in either summer or winter periods.
Remarks
The member functions return a reference to the element of the controlled sequence at position off. If that position is invalid, the behavior is undefined.
There is also a non-member get function available to get a reference to an element of an array.
Example
array::operator=
Replaces the controlled sequence.
Parameters
right
Container to copy.
Remarks
The member operator assigns each element of right to the corresponding element of the controlled sequence, then returns *this
. You use it to replace the controlled sequence with a copy of the controlled sequence in right.
Example
array::pointer
The type of a pointer to an element.
Remarks
The type describes an object that can serve as a pointer to elements of the sequence.
Example
array::rbegin
Designates the beginning of the reversed controlled sequence.
Remarks
The member functions return a reverse iterator that points just beyond the end of the controlled sequence. Hence, it designates the beginning of the reverse sequence.
Example
array::reference
The type of a reference to an element.
Remarks
The type describes an object that can serve as a reference to an element of the controlled sequence.
Example
array::rend
Designates the end of the reversed controlled sequence.
Remarks
The member functions return a reverse iterator that points at the first element of the sequence (or just beyond the end of an empty sequence)). Hence, it designates the end of the reverse sequence.
Example
array::reverse_iterator
The type of a reverse iterator for the controlled sequence. Traktor pro 3 torrent.
Remarks
The type describes an object that can serve as a reverse iterator for the controlled sequence.
Example
array::size
Counts the number of elements.
Remarks
The member function returns N
.
Example
array::size_type
The type of an unsigned distance between two element.
Remarks
The unsigned integer type describes an object that can represent the length of any controlled sequence. It is a synonym for the type std::size_t
.
Example
array::swap
Swaps the contents of this array with another array.
Parameters
right
Array to swap contents with.
Remarks
The member function swaps the controlled sequences between *this
and right. It performs a number of element assignments and constructor calls proportional to N
.
Since it is preinstalled, you should be able to read Gujarati on Internet web pages. Another Windows font that includes Gujarati characters is Arial Unicode MS that comes with Microsoft Office. However, you will not be able to type with it until you install Gujarati language support in Windows. This font is universal because it includes multiple languages; therefore it is more than 10mb in size. Download shruti.ttf for android phone.
There is also a non-member swap function available to swap two array instances.
Example
Cbegin Cend Dev C 4
array::value_type
Dev C++ Online
The type of an element.
Remarks
The type is a synonym for the template parameter Ty
.